With the last post on Xamarin Forms we went over using GZip Compression with Xamarin Forms which helps reduce data going over mobile networks. In this post, we’ll be looking at how to perform some simple animations with Xamarin Forms.
At RedBit we have Kevin, our lead designer, who makes our software and customer software usable and visually appealing. When envisioning and designing a project he suggests subtle animations to provide within the app. Then as a team, along with the customer, decide on what works and what doesn’t and then implement it.
In this article we are going to look at simple animations and sliding a panel in and out from the right side of the screen using Xamarin Forms. This is the end result of this article
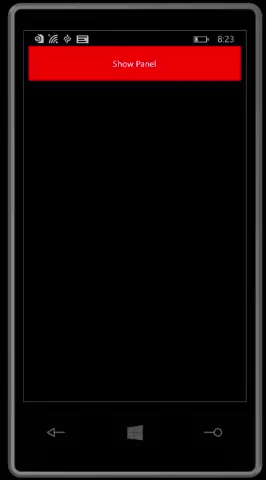
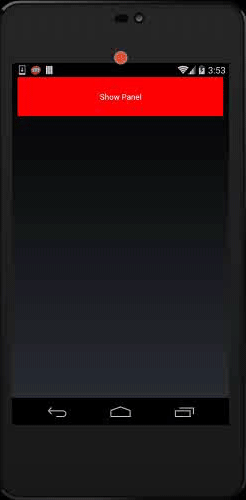
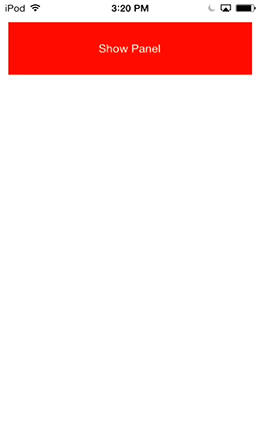
If you want to skip all the reading and want to go straight to the code, you can download it from GitHub under the RedBit.Samples.Animations repo. If you want all the background read on!
Animations by Platforms
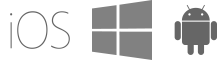
There are different types of animations and transitions that can be accomplished on each platform and each platform has their own APIs of creating these.
For Windows, you are using Storyboards and use a combination of XAML and C#. You can get a good overview in the article on MSDN Animating Your UI.
For iOS, there is obviously a different set of APIs available and are dealing with UIKit and Core Animations. With UIKit you are animating properties of UIViews with methods such as animateWithDuration and is easier from a developer perspective. Core Animations provides finer control but can be a bit more complex to get going. For more details on animations on iOS see Animations from iOS Developer Library and also Graphics & Animation in iOS from Xamarin.
On Android, again there is an entirely different set of APIs because we are on a different platform. With Android, animation options are Property Animations, View Animations and Drawable Animations with the preferred method being Property Animations as it provides more features and flexibility. For an overview, see the Android Developer Docs Animation Overview and Graphics & Animation in Android from Xamarin.
Animations with Xamarin Forms

Now that there is an understanding of how animation is accomplished and complexity levels on the different platforms, we can look at animations using Xamarin Forms.
Now to set expectations, don’t expect Xamarin forms to do overly complex animations and you may find it difficult to do some animations found on sites like CAPPTIVATE.co. If you are looking for overly complex animations, Xamarin Forms is not for you. But if you are looking for subtle animations and transitions, then Xamarin Forms should fit your needs.
Also, for the examples that have been built, we are using RelativeLayout and have not tested with other types of layouts.
Now that expectations are set, we are going to look at two examples which are animating a button to have a ‘press effect’ and sliding in a panel to show transitions.
Xamarin Forms View Extensions
To make animations easy and cross platform, Xamarin Forms provides two extension classes
The ViewExtensions class provides extension methods to easily perform animations. Within ViewExtensions you have methods such as CancelAnimations, FadeTo, LayoutTo, ScaleTo plus others.
AnimationExtensions provides methods that give developers more control on how the animations occur and Actions and Funcs as callbacks for handling completions or repeating animations.
Now that you have some background on animations using Native APIs and how extension methods in Xamarin forms, let’s look at actually making some animations in code.
Animated Button
The first animation we are going to look at is animating a button so when the user taps on it, there is a press effect to give some visual feedback for the interaction with the element.
To accomplish this we will create a custom class called AnimatedButton inheriting from ContentView. Within the AnimatedButton a TapGestureRecognizer is used to run the animations and the core code to run the animation is as follows
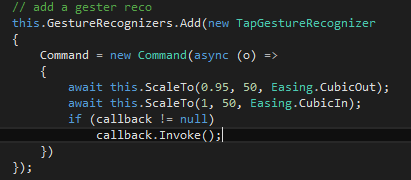
Notice how we call and await the ScaleTo method and essentially scale down to 0.95 and then scale back up to 1.
Then we will need to create a method to create the button and call the method from the contructor as follows

Run the application on iOS and you will get a result as follows
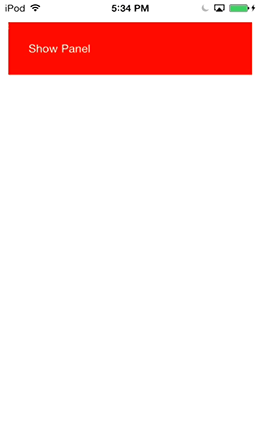
Running it on Windows Phone and Android produces the same results

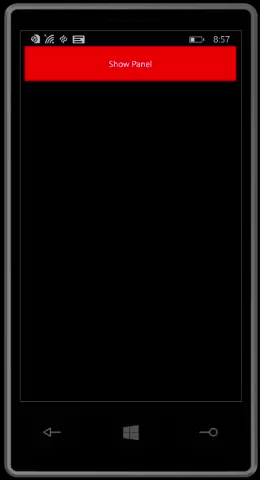
Animated Panel
Now that we have a button we can add code to show a panel to slide out from the right side. The end result will be as follows on Windows Phone
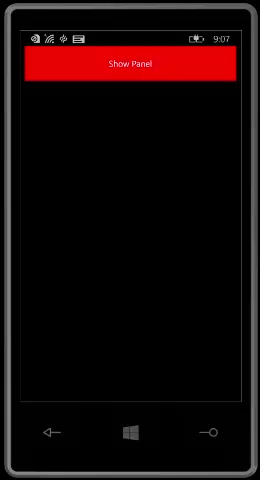
First thing we need to do is create the panel so add the following code to MainPage.cs and call the method from the constructor. Remember you can download the code from GitHub if you don’t want to type it all out.
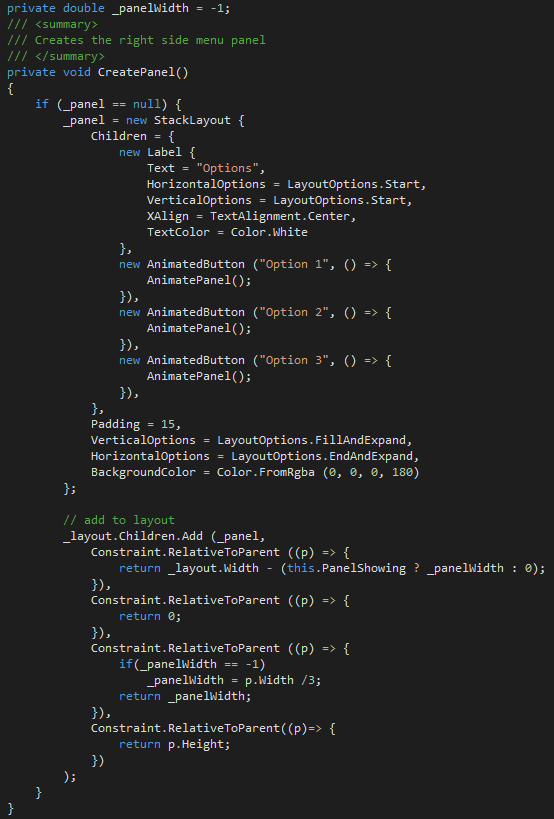
Important items to note here
- When we add the panel to the to the _layout variable, we store the original width of the panel so we can reuse it. This is primarily to support rotating the screen
- Setting the Y constraint, we either set it to the width of the _layout or width of _layout subtract the width of the panel depending what state we are in
Next, we need to update the ‘Show Panel’ animated button to show or hide the panel as required. We do this by passing in an Action to the creation of the AnimatedButton in the CreateButton method as follows
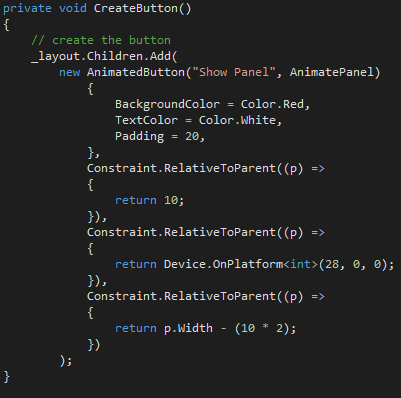
When that is done, we need to add an AnimatePanel method which will animate the panel in by sliding it in from the right into view if it’s not visible and out of view if it is visible. We will also add a property to set the state of the panel.

Deploy and run the application on iOS or Android. When the button is pressed, it will slide in and out the panel similar to Windows Phone.
Adding Some Bounce
If you run the sample you will notice that the panel slides in and it is using Easing.CubicIn and Easing.CubicOut, but the menu items are pretty static. To fix this we can use the ScaleTo extension method for the panel items. To accomplish this, we change the AnimatePanel implementation to the following
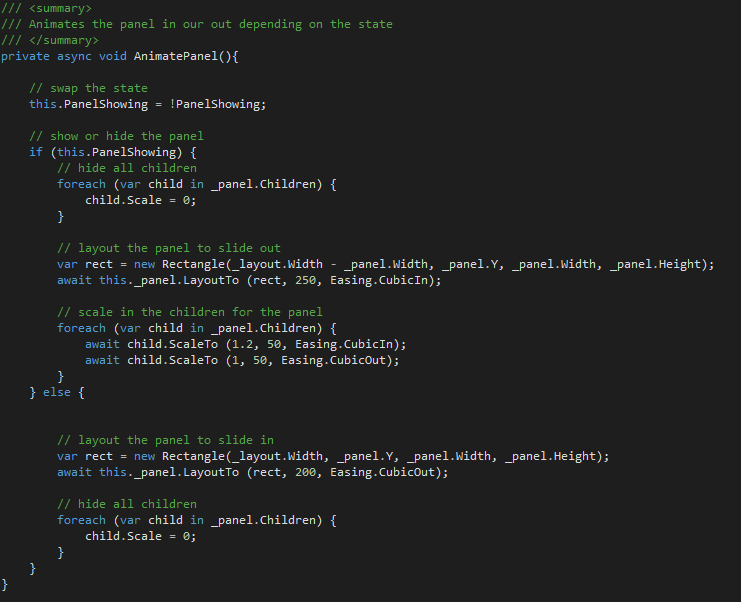
This results in a nice ‘bounce’ effect for the menu items looking as follows on the different platforms.
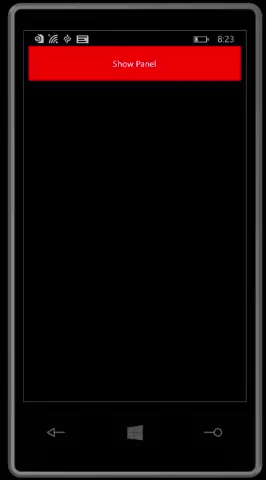
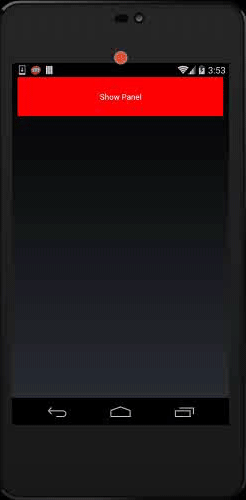
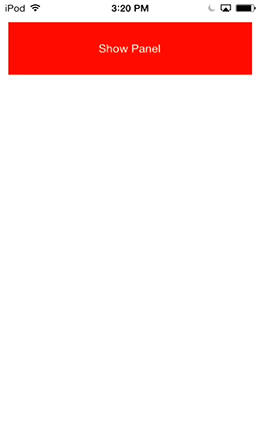
Custom Animations
Using AnimationExtensions you have a bit more control on how animations are handled and the capabilities to change properties of an element for example BackgroundColor of a Layout. You also have the opportunity to change multiple elements on the screen.
One major difference is, with AnimationExtensions you don’t have the convenience of async/await and are using callbacks instead.
In the following example, we are just going to change the BackgroundColor property of the _layout with the following code

Notice here how we are changing the background color from White to Black and back to White by setting the BackgroundColor property of our RelativeLayout. We can affect the animation by using the various params for Animate method such as the length of time it runs.
To run the code, call the ChangeBackgroundColor method when Option 1 is clicked on the slide out panel (should be around line 72).
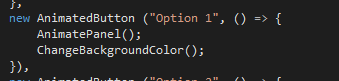
Run the sample on either iOS, Android or Windows and you will get something as follows
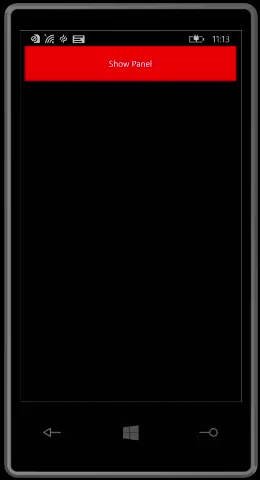
More Complex Animations
Using Xamarin Forms, you can definitely perform transitions and animations that are more complicated, but you will have to create some custom renderers. That is beyond the scope of this article but see the Xamarin developer docs for more information on custom renderers. I will leave that as an exercise for you to explore this functionality.
Wrapping Up
So to wrap up this article, you can accomplish some pretty simple cross platform animations using Xamarin Forms by using ViewExtensions or AnimationExtensions. If you require transitions and animations that are more complex, you will have to create custom renderers or opt out of using Xamarin Forms and use Xamarin.iOS or Xamarin.Android directly.
As always, all the code is available on GitHub at RedBit.Samples.XForms.Aniamtions and feel free to leave your comments or questions here or on GitHub.